Table of Contents
Install and Configure Firebase Analytics package
Hello, there.
You’re building two mobile apps (iOS and Android) using React Native. The apps get approved in their stores. How do you know if your customers are enjoying your creation and find it useful? You don’t unless you find a way to get insights and understand how your apps are used.
First, one has to decide what library should be used. Fortunately, there are some great choices that make it easy to integrate, like:
- AWS Amplify’s analytics
- Firebase analytics: In this article, I’ll be focusing on Firebase analytics, as it’s one of the most popular — especially because of its easy integration with Google Analytics. Love it or hate it, Google services are still (arguably) the most popular ones for many valid reasons.
Configure Firebase Account
Before integrating Firebase for React Native, you need a Firebase project in the console. This project will hold information for both iOS and Android apps. In my case, I named the project tutorial-demo
.
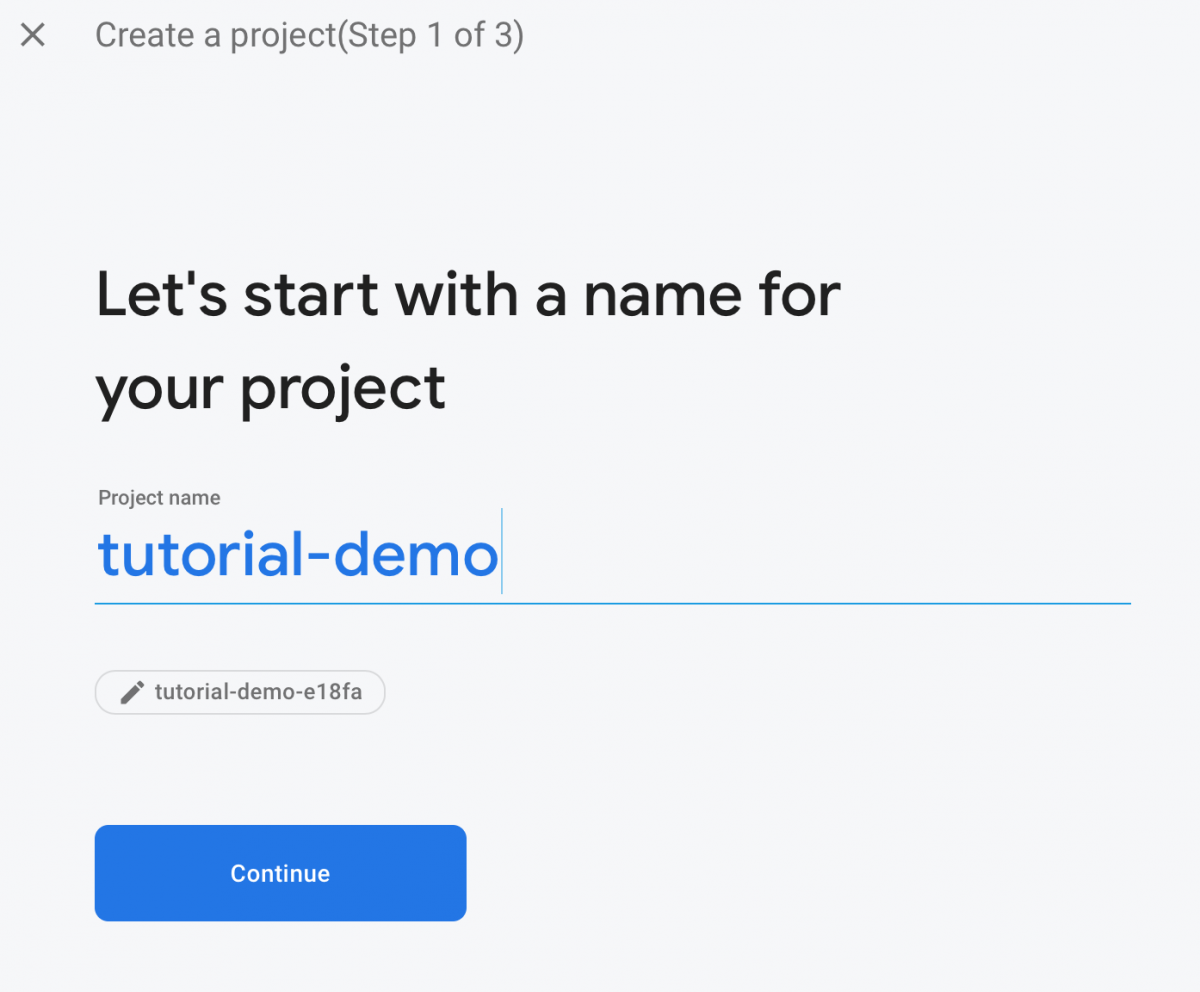

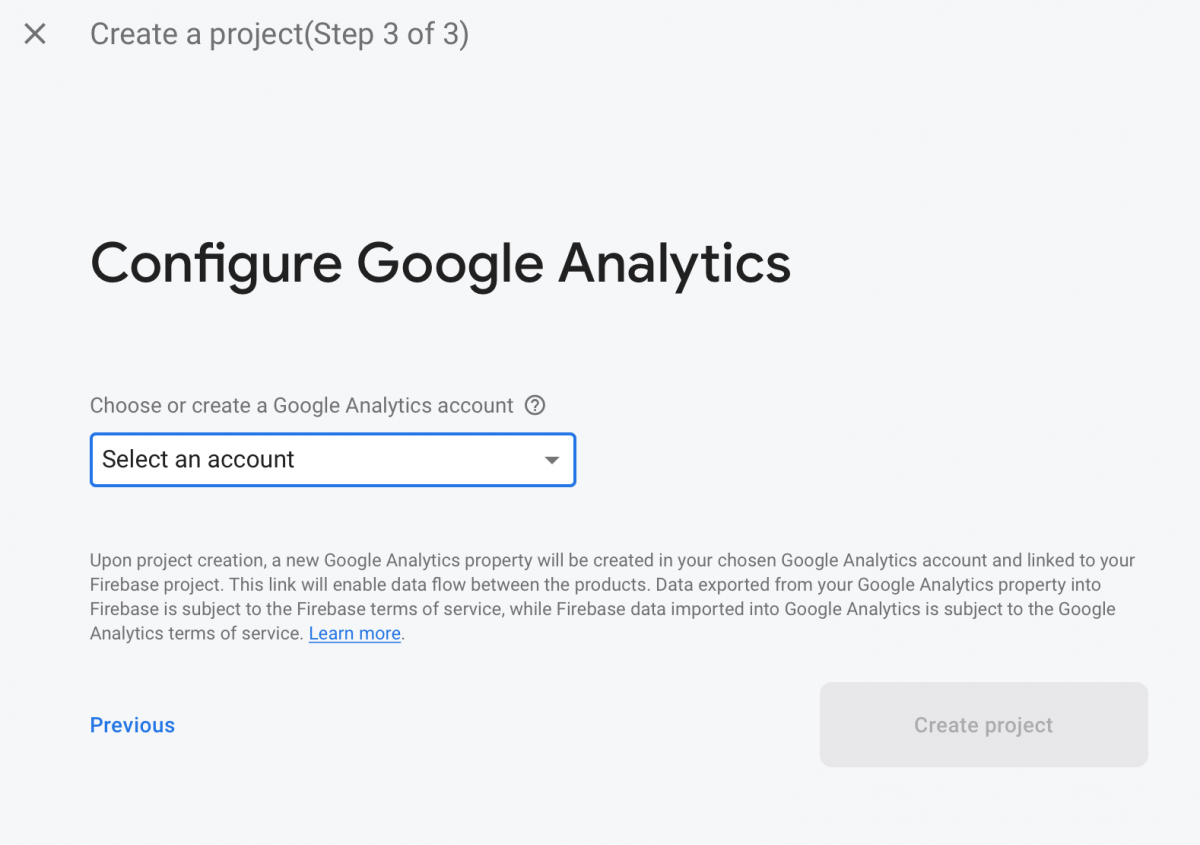
iOS project
After getting through the wizard, select the newly created project.

Now, we need to add different apps for different platforms. For iOS, select the iOS icon. Enter the native project’s bundle ID (and App Store ID, if you have one), give it a nickname, and press “Register app.”


The Firebase console provides a GoogleService-Info.plist
file. This contains a set of credentials for iOS devices to use when authenticating with your Firebase project.
Download the GoogleService-Info.plist
presented in the second step, and add it to the iOS native project. Don’t forget to select the correct target if you’re having multiple targets. Also, don’t forget to select “Copy items if needed.”


Note: Open GoogleService-Info.plist
, and enable analytics by setting “YES.” The key is IS_ANALYTICS_ENABLED
.

The third step isn’t relevant for us, as we’re covered by the Firebase package that’ll add the pods for us. The fourth step is something we can add later. For now, let’s finish with the Firebase console configurations.
Android project
The Android side of things is very similar. Go to the project homepage, and select the Android icon.
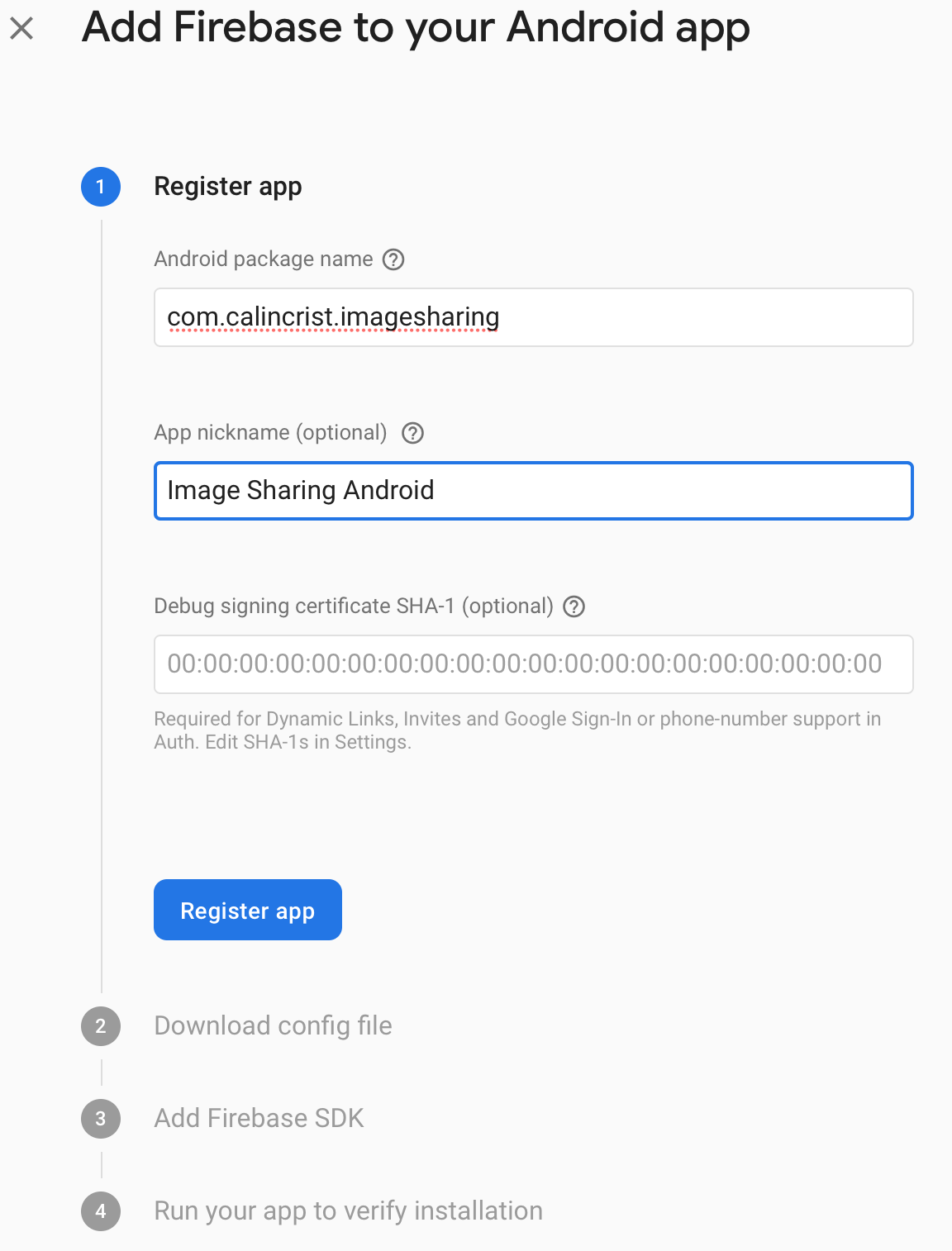
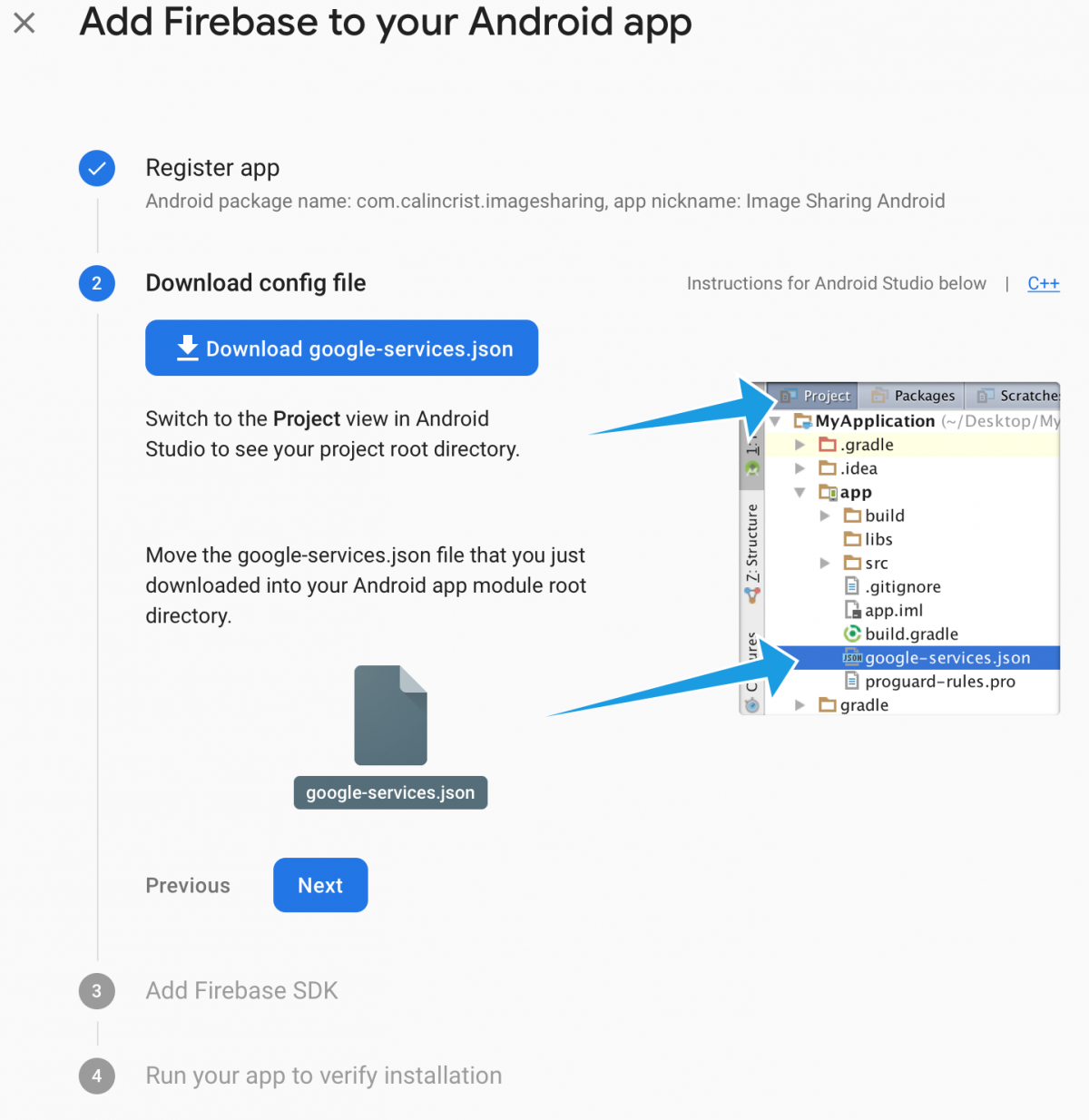
Here, we have a config file again — this time called google-services.json
. Add it to the native project inside the app
folder from the Android project folder.
Install and Configure Firebase Analytics package
For React Native, there’s the official Firebase package. It contains all of the Firebase services, and we’ll be installing and using the one. Install the core and analytics packages.
Assuming the React Native version is >= 0.60, the module should be automatically linked to your project. If not, you need to manually integrate the app
module into your project.
See the following steps for Android and iOS for more information on manual linking. Install the pods for the iOS app: cd ios && pod install && cd
For iOS, I noticed that after integrating the Firebase package, I needed to do some extra steps to make it work:
- Clear the derived data
- Clean the project
- Remove the existing app from the simulator/testing device
For Android, in case the build or the gradle syncing is failing — it happened to me on one occasion — this is what I modified. I think it has to do with autolinking failing for some reason. And here’s android/app/build.gradle.
Analytics layer
Automatic events
Just by integrating the Analytics package, there are some events that are collected automatically like: first_open
, user_engagement
, app_clear_data
. More details are provided here.
Custom events
What’s cool about this package is it provides predefined methods for different use cases depending on the nature of your app (e-commerce, games, etc.) but also bare-bones functions to customize your own event logging. Long story short, what we can do using react-native-firebase
is:
Behind the scenes, these specific events (logAppOpen
, logLogin
, logSignUp
) are using the logEvent
method specifying the key and some properties for you.
It’s highly recommended not to send any fragile and secret data to Firebase (emails, passwords, names, etc.) — not even hashed.
And these are just a bunch of them. Here are all the supported methods.
Integrating it in your project and use cases
Now to demo these events: Let’s do an old-fashioned class that’ll be used to centralize the analytics code. An advantage of this approach would be that we can use multiple analytics solutions/packages by updating just one file. (Of course, it doesn’t need to be a class but here we are.)
Track Sign ins
What’s left is to use our analytics static methods where they belong in the code.
Tracking screens
Here, we have multiple options, depending on what do we need. Either track them separately in each component after they’re being mounted or make use of events other packages we might have in our project. One example could be the beloved and frequently used react-navigation
.
Custom events
An example would be to track an image-sharing app: Either the users are more inclined to use the camera or the camera roll.
Last step: see it working
Everything is installed, configured, and implemented. Let’s see if we get something from the app to the Firebase console. Nothing? Well, there’s a delay of about one hour between logging and seeing the events on the dashboard. The good news is there’s something we can do to test it quickly — with a latency of about one second. It’s called DebugView
.
iOS
For the iOS project, we can pass an argument on the run
process by editing the scheme. The argument is called -FIRDebugEnabled
.

For the release builds, we should specify the argument-FIRDebugDisabled
.
Android
To enable debug mode on Android, just run: adb shell setprop debug.firebase.analytics.app package_name
. This behavior persists until you explicitly disable debug mode by specifying the following command-line argument: adb shell setprop debug.firebase.analytics.app .none
. Now run the apps again, and you should see some action in the Firebase console:

From my experience: if for some mystical reason it doesn't work on iOS, what did the trick for me is to manually link the libraries in Xcode, like in the picture below.
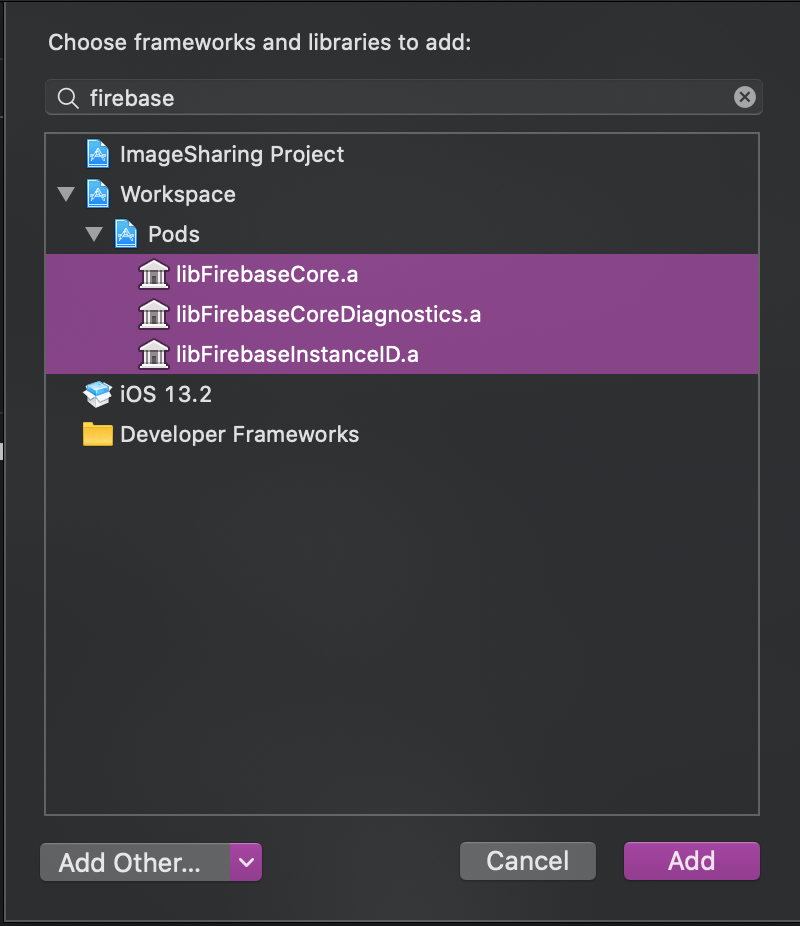
Conclusion
And that's pretty much it. What you can do from here is release your app and gather valuable information. Pair it with data from Google Analytics and you have the power (and the data to back it up) to decide what's the best next move.
For the full code here's the Github link: https://github.com/calincrist/imageSharingApp.
Thank you for reading! If you enjoyed this post and want to explore topics like this, don’t forget to subscribe to the newsletter. You’ll get the latest blog posts delivered directly to your inbox. Follow me on LinkedIn and Twitter/X. Your journey doesn’t have to end here. Subscribe, follow, and let’s take continue the conversation. This is the way!